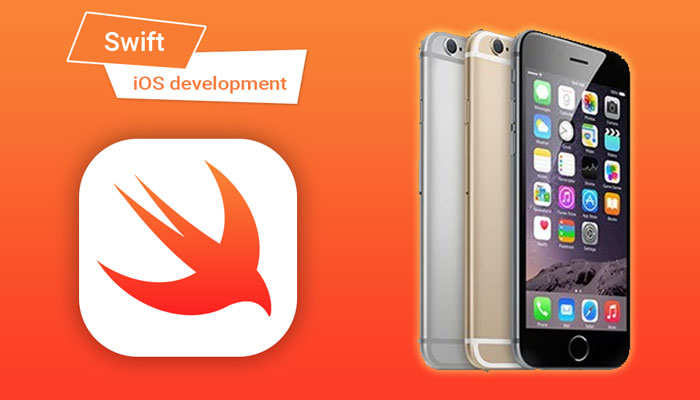
Introduction to iOS Development with Swift :
- Overview of iOS platform and its architecture
- Setting up Xcode IDE for Swift development
- Understanding the structure of an iOS project in Swift
- Creating a simple "Hello World" app and running it on the iOS simulator
User Interface Design with Interface Builder and Swift :
- Introduction to Interface Builder for designing user interfaces visually
- Working with UIKit components: UILabel, UITextField, UIButton, etc.
- Auto Layout: Creating responsive layouts for different screen sizes
- Responding to user interactions with UIKit controls and event handling in Swift
View Controllers and Navigation :
- Understanding the concept of view controllers in iOS
- Creating multiple view controllers and navigating between them
- Passing data between view controllers using segues and delegates
- Customizing navigation controllers and implementing tab bar navigation
Data Storage and Retrieval :
- Working with Core Data framework for local data storage
- Implementing CRUD (Create, Read, Update, Delete) operations with Core Data
- Using UserDefaults for storing simple data preferences
- Introduction to Codable protocol for encoding and decoding data
Networking and Web Services :
- Making network requests using URLSession framework
- Parsing JSON data from web services using Codable protocol
- Implementing RESTful APIs with URLSession and Codable
- Handling asynchronous tasks with Grand Central Dispatch (GCD) or URLSession data tasks
Final Project :
- Final project: Students will apply their knowledge to build a complete iOS app using Swift, incorporating user interface design, data storage, networking, and integration with device features